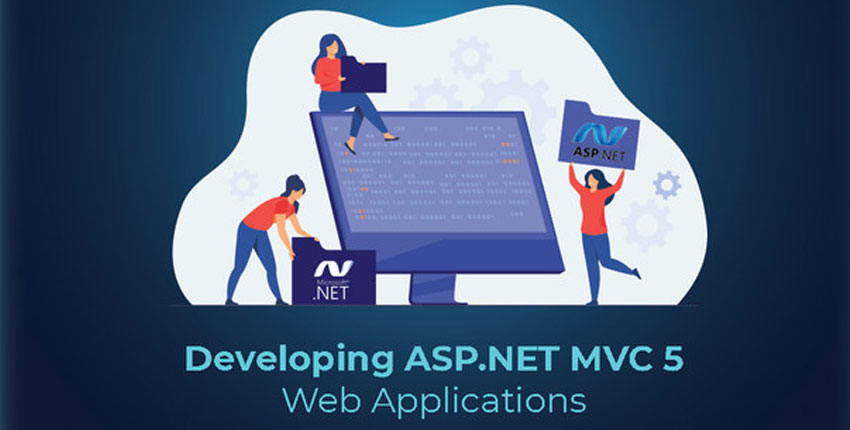
Module 1: Exploring Core ASP.NET MVC 5
- Overview of Microsoft Web Technologies
- Overview of ASP.NET
- Introduction to ASP.NET MVC 5
- Exploring a Photo Sharing Application
- Exploring a Web Pages Application
- Exploring a Web Forms Application
- Exploring an MVC Application
Module 2: Designing ASP.NET Core MVC 5 Web Applications
- Planning in the Project Design Phase
- Designing Models, Controllers, and Views
- Planning Model Classes
- Planning Controllers
- Planning Views
- Architecting an MVC Web Application
Module 3: Developing Core ASP.NET MVC 5 Models
- Creating MVC Models
- Working with Data
- Creating an MVC Project and Adding a Model
- Adding Properties to MVC Models
- Using Data Annotations in MVC Models
Module 4: Developing ASP.NET Core MVC 5 Controllers
- Writing Controllers and Actions
- Writing Action Filters
- Adding an MVC Controller and Writing the Actions
Module 5: Developing ASP.NET Core MVC 5 Views
- Creating Views with Razor Syntax
- Using HTML Helpers
- Re-using Code in Views
- Adding a View for Photo Display
- Adding a View for New Photos
- Creating and Using a Partial View
- Adding a Home View and Testing the Views
Module 6: Testing and Debugging ASP.NET Core MVC 5 Web Applications
- Unit Testing MVC Components
- Implementing an Exception Handling Strategy
- Performing Unit Tests
- Optional – Configuring Exception Handling
- Analyzing Information Architecture
- Configuring Routes
- Creating a Navigation Structure
- Using the Routing Engine
Module 7: Applying Styles to ASP.NET Core MVC 5 Web Applications
- Using Layouts
- Applying CSS Styles to an MVC Application
- Creating an Adaptive User Interface
- Creating and Applying Layouts
- Applying Styles to an MVC Web Application
Module 8: Building Responsive Pages in ASP.NET MVC 5 Web Applications
- Using AJAX and Partial Page Updates
- Implementing a Caching Strategy
- Using Partial Page Updates
Module 9: Using JavaScript and jQuery for Responsive MVC 5 Web Applications
- Rendering and Executing JavaScript Code
- Using jQuery and jQuery
- Creating and Animating the Slideshow View
- Optional—Adding a jQuery-UI Progress Bar Widget
Module 10: Controlling Access to ASP.NET MVC 5 Web Applications
- Implementing Authentication and Authorization
- Assigning Roles and Membership
- Configuring Authentication and Membership Providers
- Building the Logon and Register Views
- Authorizing Access to Resources
Module 11: Building a Resilient ASP.NET MVC 5 Web Application
- Developing Secure Sites
- State Management
- Creating Favorites Controller Actions
- Implementing Favorites in Views
Module 12: Implementing Web APIs in ASP.NET MVC 5 Web Applications
- Developing a Web API
- Calling a Web API from Mobile and Web Applications
- Adding a Web API to the Photo Sharing Application
- Using the Web API for a Bing Maps Display
Module 13: Handling Requests in ASP.NET MVC 5 Web Applications
- Using HTTP Modules and HTTP Handler
- Creating a Photo Chat View
Module 14: Adding Bootstrap/jQuery in ASP.NET MVC 5 Web Applications
- Deploying a Web Application in Bootstrap Studio.
- Deploying an ASP.NET MVC 5 Web Application.
- Adding Bootstrap Styles.
- Adding Sidebars.
- Adding Dropdown.
- Adding List.
- Adding Navbar.
- Adding jQuery plug in ASP.NET MVC 5 Web Applications
Using Basic Functions in MySQL
- Use GROUP BY and HAVING clause in MySQL queries
- Use the Mathematical functions
- Use the date functions
- Use String information function
- Use the system information functions
Controlling and Managing MySQL database
- Create User Account
- Allocate appropriate privileges to users
- Alter privileges
- Using GRANT command to assign privileges to user
- Using REVOKE command to alter privileges granted to users