This course introduces computer programming using the C# programming language with object oriented programming principles. Emphasis is placed on event-driven programming methods, including creating and manipulating objects, classes, and using object-oriented tools such as the class debugger. Upon completion, students should be able to design, code, test, debug, and implement objects using the appropriate environment at the beginning level.
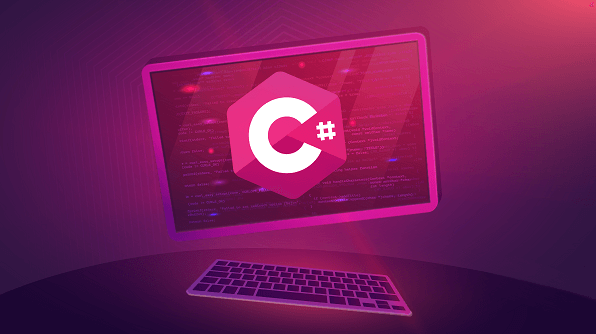
Learning Outcomes
Upon completion of this course, the student will be able to:
- Read, write, execute, and debug C# applications
- Understand variables and datatypes
- Code decision and control structures (if, if/else, switch, while, do/while, for) and use primitive data types
- Write user-defined methods
- Write and manipulate arrays
- Write programs using object-oriented programming techniques including classes and objects
- Inheritance and polymorphism
- Exception Handling
- Events and Delegates
- Collections and Generics
- File Mamagement.
Basic Concepts – Getting started with C#
- Describe the .NET Framework
- List the other components of .NET Framework
- Explain CLR and MSIL
- Define Memory Management and Garbage Collection
- Describe the Microsoft Visual Studio 2008 Product
- Learn the key elements of Visual Studio 2008 IDE
- List the basic features of C#
Variables and Data types
- Identify basic data types in C#
- Explain XML source code documentation
- List the keywords in C#
- List and explain the escape sequence characters
- Describe console output methods in C#
- Explain date and time format specifiers
- Logical operators
- Bit-wise operators
- Understand the precedence and order of evaluation
- Discuss mixed mode expressions and implicit type conversations
- Indentify C++ shorthands
Statements and Operators
- List the statement categories in C#
- Identify and explain the use of Arithmetic operators
- Identify and explain the use of relational, logical and conditional operators
- Explain Boxing and Unboxing
C# Programming Constructs
- State the syntax and working of switch….case statement
- State the syntax and working of while loop
- State the syntax and working of for loop
- Describe the goto statement
- Describe the break and continue statements
Arrays
- State the syntax of declaring arrays
- Describe Array class and its purpose
- List the commonly used properties and methods in Array class
Classes and Methods
- Explain Object-Oriented Programming
- Describe static methods
- List the types of access modifiers
- Identify and explain ref and out keywords
- Describe method overloading
- Explain constructors in C#
- Describe the use of garbage collector in C#
Inheritance and Polymorphism
- Explain the concept of inheritance
- Define sealed classes in C#
- Explain how to implement polymorphism
Abstract Classes and Interfaces
- Describe how to implement abstract classes
- Define abstract methods
- Describe how to implement interface
- List the differences between abstract classes and interfaces
Properties and Indexers
- Define properties in C#
- List and explain the types of properties
- State and explain indexers
Namespaces
- Describe namespaces and their purpose
- Describe custom namespaces
- Explain namespace aliases
Exception Handling
- Describe Exceptions in C#
- List the commonly used exception classes
- Describe custom exceptions and their purpose
Events and Delegates
- Explain the concept of delegates
- Describe delegates in C#
- Explain how to use a delegate in a C# application
- Explain the steps to create and use events
Collections
- List and explain the commonly used classes and interfaces in the
- Systems.Collections namespace
- List and explain the commonly used classes and interfaces in the
- Systems.Collections.Generic namespace
- Describe the commonly used methods and properties of ArrayList class
- Describe the commonly used methods and properties of Hashtable class
- Describe the commonly used methods and properties of SortedList class
- Describe the commonly used methods and properties of Dictionary generic class
Generics and Iterators
- Explain the concept of generics in C#
- Explain the System.Collections.ObjectModel namespace
- State the syntax of creating a generic class
- Describe iterators in C#
- Explain how to use iterators
Anonymous Methods, Partial Types and Nullable Types
- Explain how to pass parameters to anonymous methods
- Explain how to implement partial types
- Explain how to implement nullable types
Debug, Test and Deploy Applications
- Discuss how to debug and troubleshoot applications
- Discuss how to test C# applications
- Discuss deployment concepts
- Discuss deploying of applications
- Explore deployment alternatives
- Create a sample application, package and deploy it