This course of study builds on the skills gained by students in Java Fundamentals and helps to advance Java programming skills. Students can design object-oriented applications with Java and will create Java programs using hands-on, engaging activities.
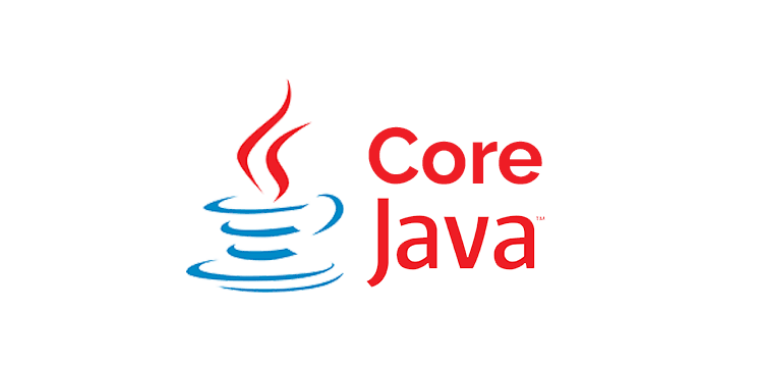
Learning Outcomes
At the end of the course the participant will:
- Implement Object Oriented Programming Concepts
- Use and create packages and interfaces in a Java program
- Use graphical user interface in Java programs
- Create Applets
- Implement exception handling in Java
- Implement Multithreading
- Use Input/Output Streams
- Handle security implementations in Java
Introduction to Java
- Creation of Java
- Features of Java
- Overview of Programming with JDK
- Discuss the Java Security Model
- Describe Java Virtual Machine
- Garbage Collection and Memory Management
- Sample Programs
Object Oriented Programming
- Structures Programming technique
- Object Oriented Programming and its advantages
- Define Objects and Classes and the relation between them
- Explain terms-Attributes, Methods, Construction, Destruction and Persistence
- Discuss Data Abstraction
- Discuss Data Encapsulation
- Explain Polymorphism
- Explain Inheritance
Basics of Java
- Discuss the data types available in Java and utilize them in applications
- Describe the various control structures and loops available in Java
- Explain and utilize the various operators present in Java
- Explain an Array
Methods and Classes
- Describe class and inheritance of classes
- Explain and Object and to declare one
- Explain the concept of Constructors and its relation with respect to class
- Methods
- “this” keyword
- Concept of Inner class
Packages and Interfaces
- Purpose of Modifies and its types
- Explain Package
- List the various Packages available in Java
- Design user defined Package
- Explain the term interface and how to implement it
- Practice creating an interface and implement it
String and String Buffer
- String Class
- Various Methods of the String class and how to use them
- Explain StringBuffer class
- Discuss the methods of the StringBuffer class and its usage
Java Applets
- Difference between Applications and Applets
- Understand the Applet Class
- Create, run and execute Applets
- Understand the Security Restrictions applied on Applet
- Identify the various activities in an applet
- Identify how to use Components and layouts in Applets
Graphics
- Basics of Graphic System
- Explain and utilize the following geometric figures in applications
- Lines
- Ovals
- Rectangle and RoundRectangle
- 3DRectange and Arc
- Discuss Color Control and how to use it to fill color in the container and the images in it
- Disuss the Font Control and how to play around with it
- Explain the FontMetrics class and its purpose
Basic Graphical User Interface Components (Abstract Window ToolKit (AWT)
- Describe the concept of GUI
- Discuss the following Handle events using the following listeners
- ActionListener
- ItemListener
- WindowListener
- ComponentListener
- MouseListener
- MouseMotionListener
- Describe the following components and how to apply them in the container
- Label
- List
- Button
- Checkbox
- Choice
- TextField
- TextArea
- FileDialog
Advanced GUI Components
- Discuss event handling related to mouse events
- Describe the LayoutManager class and implement the types of layout in the application
- Discuss the various containers and how to implement them
- Understand Menus and apply them in the frame
Exception Handling
- Discuss the purpose of Exception Handing in Java
- Explain the types of exception in Java
- Describe the use of try and catch
- Explain the use of throws keyword
- Explain the purpose of throw
- Describe the finally keyword
Multithreading
- Describe Multithreading
- Creating and Managing Threads
- Discuss the life cycle of threads
- Understand the concept of synchronization
- Explain how to set the priorities of thread
- Understand what a daemon thread does