This course of study builds on the skills gained by students in Java Fundamentals and helps to advance Java programming skills. Students can design object-oriented applications with Java and will create Java programs using hands-on, engaging activities.
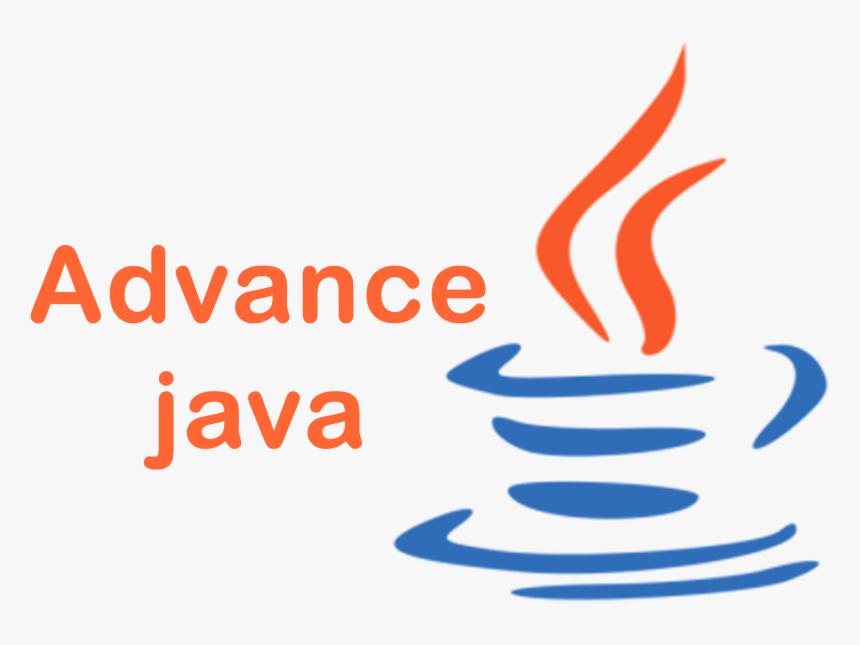
Swing Programming (Graphical User Interface)
- Understand difference between Swing and AWT programming
- Define Swing components
- List Swing Packages
- List the sub-classes of the JComponent class
- Explain how swing handles events
- Use JFC to write swing applets
- Understand the concept of “Look and Feel”
SQL Database
- SQL Commands
- Data Definition (DDL) Commands
- Data Manipulation (DML) Commands
- Transaction Control (TCL) Commands
- Aggregate Functions
JDBC(Java Database Connectivity)
- Steps to connect java and Database
- SQL Statements
- Use JDBC to access a database
- Set up a connection to the database
- Create and execute SQL Statements
- Describe the Result Set object
Servlet Technology
- Request Object
- Response Object
- Session Management
- http Session
- Cookies
- Hidden form field
- URL Rewriting
- Request Dispatcher
Hibernate
- Hibernate framework
- Introduction ORM
- Hibernate Mapping
- Hibernate One-to-One Mapping
- Hibernate Query Language (HQL)
- HQL Select Query
- HQL Update, Delete Queries
Introducing Java Server Pages
- The Java2 Enterprise Edition
- JSP
- How does JSP does its stuff
- Comparison with existing technologies
- Future of the Platform
The Basics
- What do JSP contains?
- JSP Life Cycle
- Directives
- Scripting Elements and Implicit Objects
JSP Directives
- Directive Basics
- The page Directive
- The taglib Directive
- The include Directive
JSP Standard Actions
- JSP Standard Actions Are Built-in Tags
- Actions for Working with JavaBeans
- Including JSP Output via <jsp:include>
- Transferring Control between JSPs
- Specifying Parameters for Other Actions
Beneath JSP
- Web Application Server Architecture
- Important Servlet API Features
- Session tracking
- Form data parsing
JSP and Java Beans
- Introduction
- Java Beans
- Java Beans and JDBC
- Creating Sample Application
JSP Sessions
- Persistent Connections
- Working with Cookies and JAVA
- Sessions in Action
- Sessions, HTTPS and JSP
Error Handling with JSP
- Types of Errors and Exceptions
- JSP Specific Exception Classes
- An Example Web Application
Java Database Connectivity and Connection Pooling
- Relational Database Management Systems
- Alien Object Model
- Why all the Driver Types?
- How JSP and JDBC fit together
- The Bigger J2EE Picture
- Coding a simple JSP using JDBC
- More Advanced JDBC
- Multiple Users and the Need for the Connection Pooling
SP Tag Libraries and JSTL
- The Need for a Tag Extension Mechanism
- The Simplest tag
- JSP Standard Tag Library
- Resources
Debugging JSP
- Why Debugging JSP is so Hard
- Different Types of Errors
- Debugging Techniques
- Future Directions
JSP Architecture
- Code Factoring and Role Separation
- Architectures
- The Page-Centric Approach
- The Dispatcher Approach
- Servlets versus JSPs
JSPs and Servlets
- A JSP is a Servlet
- JSP Compilation
Java Server Faces
- Configuring a JSF Project
- Getting Started with JSF
- JSF Lifecycle
- Validating Data
- Converting Data
- Handling Events with Listeners